The Gazebo scene viewer examples allow us to visualize Gazebo simulation using the Gazebo Rendering library.
Compile and run the example
Clone the source code, create a build directory and use cmake
and make
to compile the code:
gazebo_scene_viewer
Launch Gazebo and insert Double pendulum with base
:
Launch the example to visualize the pendulum:
You can use the Tab
button to change the rendering engine.
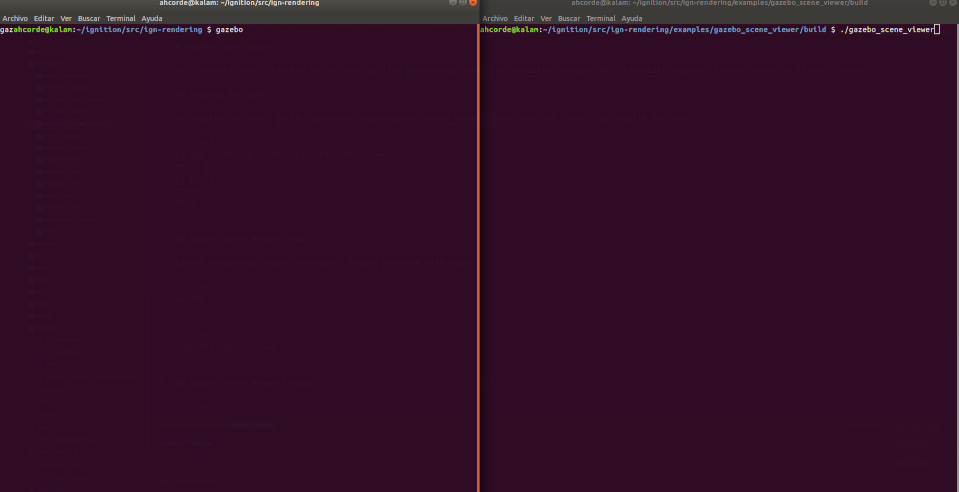
gazebo_scene_viewer2_demo
Launch Gazebo using the world inside the example directory called falling_objects.world
. You will see some objects falling.
Launch the example to visualize the objects:
You can use the Tab
button to change the rendering engine.
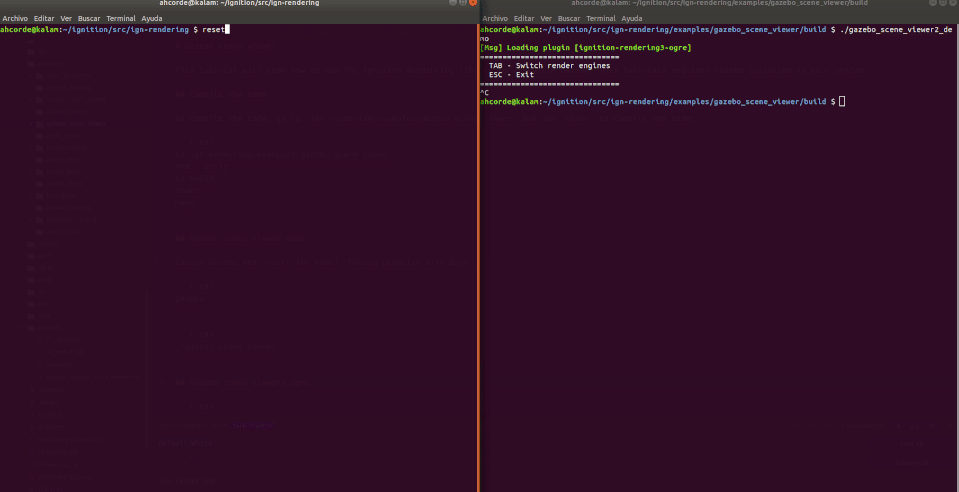
Code
The SceneManager
class defined in SceneManager.hh
, SceneManagerPrivate.hh
and SceneManager.cc
manages a collection of scenes. The class provides a single interface for modifications, allowing multiple scenes to stay synchronized. It will allow us to receive data from Gazebo and update the render window each time that the examples receive a new scene. This class currently consumes Gazebo-specific protobuf messages.
The following list will describe some methods:
- void SceneManagerPrivate::Init(): It initializes the communication with Gazebo. It will create some subscribers to receive data about poses, light, models, joints, visual or sensors.
- void SubSceneManager::ProcessMessages(): This method will process the messages received from Gazebo. Calling the right primitive to render lights, models, joints, visuals, sensors or poses of the different object received. For example, if the Gazebo scene contains a cylinder the following method will be called:this->ProcessLights();this->ProcessModels();this->ProcessJoints();this->ProcessVisuals();this->ProcessSensors();this->ProcessPoses();this->ProcessRemovals();void SubSceneManager::ProcessCylinder(const gazebo::msgs::Geometry &_geometryMsg, VisualPtr _parent){GeometryPtr cylinder = this->activeScene->CreateCylinder();const gazebo::msgs::CylinderGeom &cylinderMsg = _geometryMsg.cylinder();double x = 2 * cylinderMsg.radius();double y = 2 * cylinderMsg.radius();double z = cylinderMsg.length();_parent->SetLocalScale(x, y, z);_parent->AddGeometry(cylinder);}
- void CurrentSceneManager::OnPoseUpdate(::ConstPosesStampedPtr &_posesMsg): This method is called when the subscriber receives a new pose message. void CurrentSceneManager::OnPoseUpdate(::ConstPosesStampedPtr &_posesMsg){// record pose timestampthis->timePosesReceived = std::chrono::seconds(_posesMsg->time().sec()) +std::chrono::nanoseconds(_posesMsg->time().nsec());// process each pose in messagefor (int i = 0; i < _posesMsg->pose_size(); ++i){// replace into pose mapgazebo::msgs::Pose pose = _posesMsg->pose(i);std::string name = pose.name();this->poseMsgs[name] = pose;}}